Decompiled source of RiskOfBulletstorm v1.2.2
plugins/mod/RiskOfBulletstormRewrite.dll
Decompiled a year ago
The result has been truncated due to the large size, download it to view full contents!
using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.IO; using System.Linq; using System.Reflection; using System.Runtime.CompilerServices; using System.Runtime.Versioning; using System.Security; using System.Security.Permissions; using System.Text; using System.Text.RegularExpressions; using BepInEx; using BepInEx.Bootstrap; using BepInEx.Configuration; using BepInEx.Logging; using BetterUI; using EntityStates; using EntityStates.Barrel; using EntityStates.BrotherMonster; using EntityStates.Engi.EngiWeapon; using EntityStates.Heretic; using EntityStates.Huntress; using EntityStates.ImpMonster; using EntityStates.Interactables.StoneGate; using EntityStates.Mage.Weapon; using EntityStates.NewtMonster; using EntityStates.VagrantMonster.Weapon; using HG; using HG.Reflection; using HarmonyLib; using JetBrains.Annotations; using KinematicCharacterController; using On.EntityStates; using On.EntityStates.Interactables.StoneGate; using On.EntityStates.NewtMonster; using On.RoR2; using On.RoR2.Items; using On.RoR2.Projectile; using On.RoR2.UI; using On.RoR2.UI.LogBook; using On.RoR2.UI.MainMenu; using R2API; using R2API.ContentManagement; using R2API.ScriptableObjects; using R2API.Utils; using RiskOfBulletstormRewrite.Artifact; using RiskOfBulletstormRewrite.Characters; using RiskOfBulletstormRewrite.Characters.Enemies; using RiskOfBulletstormRewrite.Characters.Enemies.Lord_of_the_Jammed; using RiskOfBulletstormRewrite.Controllers; using RiskOfBulletstormRewrite.Equipment; using RiskOfBulletstormRewrite.Equipment.EliteEquipment; using RiskOfBulletstormRewrite.GameplayAdditions; using RiskOfBulletstormRewrite.Interactables; using RiskOfBulletstormRewrite.Items; using RiskOfBulletstormRewrite.MarkdownCreation; using RiskOfBulletstormRewrite.Modules; using RiskOfBulletstormRewrite.Utils; using RiskOfBulletstormRewrite.Utils.Components; using RoR2; using RoR2.CharacterAI; using RoR2.ExpansionManagement; using RoR2.Orbs; using RoR2.Projectile; using RoR2.Skills; using RoR2.UI; using RoR2.UI.LogBook; using RoR2.UI.MainMenu; using TMPro; using UnityEngine; using UnityEngine.AddressableAssets; using UnityEngine.Events; using UnityEngine.Networking; using UnityEngine.Rendering; using UnityEngine.SceneManagement; [assembly: CompilationRelaxations(8)] [assembly: RuntimeCompatibility(WrapNonExceptionThrows = true)] [assembly: Debuggable(DebuggableAttribute.DebuggingModes.Default | DebuggableAttribute.DebuggingModes.DisableOptimizations | DebuggableAttribute.DebuggingModes.IgnoreSymbolStoreSequencePoints | DebuggableAttribute.DebuggingModes.EnableEditAndContinue)] [assembly: OptIn] [assembly: TargetFramework(".NETStandard,Version=v2.0", FrameworkDisplayName = ".NET Standard 2.0")] [assembly: AssemblyCompany("RiskOfBulletstormRewrite")] [assembly: AssemblyConfiguration("Debug")] [assembly: AssemblyDescription("Mod for Risk of Rain 2")] [assembly: AssemblyFileVersion("1.0.0.0")] [assembly: AssemblyInformationalVersion("1.0.0+57103ff3428b0dc36cc19fb920906dd908a6c951")] [assembly: AssemblyProduct("RiskOfBulletstormRewrite")] [assembly: AssemblyTitle("RiskOfBulletstormRewrite")] [assembly: SecurityPermission(SecurityAction.RequestMinimum, SkipVerification = true)] [assembly: AssemblyVersion("1.0.0.0")] [module: UnverifiableCode] namespace RiskOfBulletstormRewrite { public static class Commands { public static byte printReadmeState; public static void Initialize() { } [ConCommand(/*Could not decode attribute arguments.*/)] public static void CCPrintReadme(ConCommandArgs _) { if (printReadmeState == 0) { printReadmeState = 1; Debug.Log((object)"Run the command again to confirm. Hooks mainmenu"); } else if (printReadmeState == 1) { ReadmeCreator.Initialization(); printReadmeState = 2; } } [ConCommand(/*Could not decode attribute arguments.*/)] public static void CCSetLayer(ConCommandArgs args) { //IL_002f: Unknown result type (might be due to invalid IL or missing references) //IL_0034: Unknown result type (might be due to invalid IL or missing references) if (((ConCommandArgs)(ref args)).GetArgInt(0) == 2001) { KinematicCharacterMotor component = ((Component)((ConCommandArgs)(ref args)).senderBody).GetComponent<KinematicCharacterMotor>(); component.CollidableLayers = LayerMask.op_Implicit(((ConCommandArgs)(ref args)).GetArgInt(1)); component.RebuildCollidableLayers(); } } [ConCommand(/*Could not decode attribute arguments.*/)] public static void CCPreviewLOTJMat(ConCommandArgs args) { //IL_0021: Unknown result type (might be due to invalid IL or missing references) //IL_0026: Unknown result type (might be due to invalid IL or missing references) if (((ConCommandArgs)(ref args)).GetArgInt(0) == 2001) { LordofTheJammedMonster.LOTJDisplayController.MaterialToSet = Addressables.LoadAssetAsync<Material>((object)((ConCommandArgs)(ref args)).GetArgString(1)).WaitForCompletion(); } } [ConCommand(/*Could not decode attribute arguments.*/)] public static void CCSpawnPrefab(ConCommandArgs args) { //IL_0023: Unknown result type (might be due to invalid IL or missing references) //IL_0028: Unknown result type (might be due to invalid IL or missing references) //IL_0039: Unknown result type (might be due to invalid IL or missing references) //IL_003e: Unknown result type (might be due to invalid IL or missing references) //IL_007d: Unknown result type (might be due to invalid IL or missing references) if (!Main.enableDebug) { Debug.LogWarning((object)"debug isn't on"); return; } GameObject val = Addressables.LoadAssetAsync<GameObject>((object)((ConCommandArgs)(ref args)).GetArgString(0)).WaitForCompletion(); Vector3 corePosition = ((ConCommandArgs)(ref args)).senderBody.corePosition; if (((ConCommandArgs)(ref args)).Count > 1) { ((Vector3)(ref corePosition))..ctor(((ConCommandArgs)(ref args)).GetArgFloat(1), ((ConCommandArgs)(ref args)).GetArgFloat(2), ((ConCommandArgs)(ref args)).GetArgFloat(3)); } GameObject val2 = Object.Instantiate<GameObject>(val); val2.transform.position = corePosition; } [ConCommand(/*Could not decode attribute arguments.*/)] public static void CCSpawnEffect(ConCommandArgs args) { //IL_0026: Unknown result type (might be due to invalid IL or missing references) //IL_002b: Unknown result type (might be due to invalid IL or missing references) //IL_003c: Unknown result type (might be due to invalid IL or missing references) //IL_0041: Unknown result type (might be due to invalid IL or missing references) //IL_0080: Unknown result type (might be due to invalid IL or missing references) if (!Main.enableDebug) { Debug.LogWarning((object)"debug isn't on"); return; } GameObject val = Addressables.LoadAssetAsync<GameObject>((object)((ConCommandArgs)(ref args)).GetArgString(0)).WaitForCompletion(); Vector3 corePosition = ((ConCommandArgs)(ref args)).senderBody.corePosition; if (((ConCommandArgs)(ref args)).Count > 1) { ((Vector3)(ref corePosition))..ctor(((ConCommandArgs)(ref args)).GetArgFloat(1), ((ConCommandArgs)(ref args)).GetArgFloat(2), ((ConCommandArgs)(ref args)).GetArgFloat(3)); } GameObject val2 = Object.Instantiate<GameObject>(val); val2.transform.position = corePosition; EffectManager.SimpleMuzzleFlash(val, ((Component)((ConCommandArgs)(ref args)).senderBody).gameObject, "HealthBarOrigin", false); } [ConCommand(/*Could not decode attribute arguments.*/)] public static void CCGetSpiceChance(ConCommandArgs _) { //IL_0018: Unknown result type (might be due to invalid IL or missing references) //IL_001d: Unknown result type (might be due to invalid IL or missing references) //IL_0022: Unknown result type (might be due to invalid IL or missing references) Run instance = Run.instance; PickupIndex pickupIndex = PickupCatalog.FindPickupIndex(EquipmentBase<Spice2>.Instance.EquipmentDef.equipmentIndex); Dictionary<string, List<PickupIndex>> dictionary = new Dictionary<string, List<PickupIndex>> { { "availableBossDropList", instance.availableBossDropList }, { "availableEquipmentDropList", instance.availableEquipmentDropList }, { "availableLunarCombinedDropList", instance.availableLunarCombinedDropList }, { "availableLunarEquipmentDropList", instance.availableLunarEquipmentDropList }, { "availableLunarItemDropList", instance.availableLunarItemDropList }, { "availableTier1DropList", instance.availableTier1DropList }, { "availableTier2DropList", instance.availableTier2DropList }, { "availableTier3DropList", instance.availableTier3DropList }, { "availableVoidBossDropList", instance.availableVoidBossDropList }, { "availableVoidTier1DropList", instance.availableVoidTier1DropList }, { "availableVoidTier2DropList", instance.availableVoidTier2DropList }, { "availableVoidTier3DropList", instance.availableVoidTier3DropList } }; StringBuilder stringBuilder = StringBuilderPool.RentStringBuilder(); foreach (KeyValuePair<string, List<PickupIndex>> item in dictionary) { int num = item.Value.Count((PickupIndex index) => index == pickupIndex); float num2 = (float)(num / item.Value.Count) * 100f; stringBuilder.AppendLine($"{item.Key} Count: {num}/{item.Value.Count} ({num2}% chance)"); } Debug.Log((object)stringBuilder.ToString()); StringBuilderPool.ReturnStringBuilder(stringBuilder); } } [BepInPlugin("com.DestroyedClone.RiskOfBulletstorm", "Risk of Bulletstorm", "1.2.2")] [BepInDependency("com.bepis.r2api", "5.0.10")] [BepInDependency(/*Could not decode attribute arguments.*/)] [NetworkCompatibility(/*Could not decode attribute arguments.*/)] public class Main : BaseUnityPlugin { public const string ModGuid = "com.DestroyedClone.RiskOfBulletstorm"; public const string ModName = "Risk of Bulletstorm"; public const string ModVer = "1.2.2"; internal static ManualLogSource _logger; public static List<ArtifactBase> Artifacts = new List<ArtifactBase>(); public static List<ItemBase> Items = new List<ItemBase>(); public static List<EquipmentBase> Equipments = new List<EquipmentBase>(); public static List<EliteEquipmentBase> EliteEquipments = new List<EliteEquipmentBase>(); public static List<ItemDef> itemDefsThatCantBeAutoPickedUp = new List<ItemDef>(); public static List<PickupIndex> pickupIndicesThatCantBePickedUp = new List<PickupIndex>(); public static string LocationOfProgram; public static PluginInfo pluginInfo; public static readonly bool enableDebug = false; public static Dictionary<ItemDef, ItemDef> voidConversions = new Dictionary<ItemDef, ItemDef>(); public static R2APISerializableContentPack ContentPack { get; private set; } public void Awake() { ContentPack = R2APIContentManager.ReserveSerializableContentPack(); } public void Start() { //IL_00a2: Unknown result type (might be due to invalid IL or missing references) //IL_00ac: Expected O, but got Unknown _logger = ((BaseUnityPlugin)this).Logger; pluginInfo = ((BaseUnityPlugin)this).Info; LanguageOverrides.config = ((BaseUnityPlugin)this).Config; AvailabilityManager.Initialize(); LocationOfProgram = Path.GetDirectoryName(((BaseUnityPlugin)this).Info.Location); Assets.Init(); SharedComponents.Init(); Buffs.CreateBuffs(); AddToAssembly(); SetupVoid(); RoR2Application.onLoad = (Action)Delegate.Combine(RoR2Application.onLoad, (Action)delegate { //IL_0019: Unknown result type (might be due to invalid IL or missing references) //IL_001e: Unknown result type (might be due to invalid IL or missing references) //IL_0023: Unknown result type (might be due to invalid IL or missing references) //IL_0035: Unknown result type (might be due to invalid IL or missing references) foreach (ItemDef item2 in itemDefsThatCantBeAutoPickedUp) { PickupIndex item = PickupCatalog.FindPickupIndex(item2.itemIndex); if (((PickupIndex)(ref item)).isValid) { pickupIndicesThatCantBePickedUp.Add(item); } } ((BaseUnityPlugin)this).Logger.LogMessage((object)((Object)CharacterBase<LordofTheJammedMonster>.Instance.BodyPrefab).name); }); ModSupport.CheckForModSupport(); Commands.Initialize(); Tweaks.Init(((BaseUnityPlugin)this).Config); LanguageOverrides.Initialize(); GenericPickupController.OnTriggerStay += new hook_OnTriggerStay(GenericPickupController_OnTriggerStay); if (enableDebug) { Run.onRunStartGlobal += Run_onRunStartGlobal; if (ReadmeCreator.isEnabled) { ReadmeCreator.Initialization(); } } } private void GenericPickupController_OnTriggerStay(orig_OnTriggerStay orig, GenericPickupController self, Collider other) { //IL_0011: Unknown result type (might be due to invalid IL or missing references) //IL_0016: Unknown result type (might be due to invalid IL or missing references) //IL_001d: Unknown result type (might be due to invalid IL or missing references) //IL_0042: Unknown result type (might be due to invalid IL or missing references) //IL_0043: Unknown result type (might be due to invalid IL or missing references) //IL_002e: Unknown result type (might be due to invalid IL or missing references) //IL_0033: Unknown result type (might be due to invalid IL or missing references) if (NetworkServer.active) { PickupIndex pickupIndex = self.pickupIndex; if (pickupIndicesThatCantBePickedUp.Contains(self.pickupIndex)) { self.pickupIndex = PickupIndex.none; } orig.Invoke(self, other); self.pickupIndex = pickupIndex; } } private void Run_onRunStartGlobal(Run obj) { Console.instance.SubmitCmd(LocalUserManager.GetFirstLocalUser().currentNetworkUser, "no_enemies", true); Console.instance.SubmitCmd(LocalUserManager.GetFirstLocalUser().currentNetworkUser, "stage1_pod 0", true); ConsoleWindow.cvConsoleEnabled.SetBool(true); } public void SetupVoid() { //IL_0008: Unknown result type (might be due to invalid IL or missing references) //IL_0012: Expected O, but got Unknown ContagiousItemManager.Init += new hook_Init(SetupContagiousItemManager); } private void SetupContagiousItemManager(orig_Init orig) { //IL_004f: Unknown result type (might be due to invalid IL or missing references) //IL_0071: Unknown result type (might be due to invalid IL or missing references) //IL_0072: Unknown result type (might be due to invalid IL or missing references) //IL_008c: Unknown result type (might be due to invalid IL or missing references) foreach (KeyValuePair<ItemDef, ItemDef> voidConversion in voidConversions) { _logger.LogMessage((object)("Adding conversion: " + voidConversion.Key.nameToken + " to " + voidConversion.Value.nameToken)); Pair val = default(Pair); val.itemDef1 = voidConversion.Key; val.itemDef2 = voidConversion.Value; Pair val2 = val; ItemCatalog.itemRelationships[ItemRelationshipTypes.ContagiousItem] = CollectionExtensions.AddToArray<Pair>(ItemCatalog.itemRelationships[ItemRelationshipTypes.ContagiousItem], val2); } orig.Invoke(); } public void AddToAssembly() { IEnumerable<Type> enumerable = from type in Assembly.GetExecutingAssembly().GetTypes() where !type.IsAbstract && type.IsSubclassOf(typeof(ArtifactBase)) select type; foreach (Type item in enumerable) { ArtifactBase artifactBase = (ArtifactBase)Activator.CreateInstance(item); if (ValidateArtifact(artifactBase, Artifacts)) { artifactBase.Init(((BaseUnityPlugin)this).Config); } } IEnumerable<Type> enumerable2 = from type in Assembly.GetExecutingAssembly().GetTypes() where !type.IsAbstract && type.IsSubclassOf(typeof(EquipmentBase)) select type; List<string> list = new List<string>(); List<EquipmentBase> list2 = new List<EquipmentBase>(); foreach (Type item2 in enumerable2) { EquipmentBase equipmentBase = (EquipmentBase)Activator.CreateInstance(item2); if (equipmentBase.ParentEquipmentName != null) { list2.Add(equipmentBase); } else if (ValidateEquipment(equipmentBase, Equipments)) { equipmentBase.Init(((BaseUnityPlugin)this).Config); list.Add(equipmentBase.EquipmentName); } } foreach (EquipmentBase item3 in list2) { if (list.Contains(item3.ParentEquipmentName)) { item3.Init(((BaseUnityPlugin)this).Config); } } IEnumerable<Type> enumerable3 = from type in Assembly.GetExecutingAssembly().GetTypes() where !type.IsAbstract && type.IsSubclassOf(typeof(ItemBase)) select type; List<string> list3 = new List<string>(); List<ItemBase> list4 = new List<ItemBase>(); foreach (Type item4 in enumerable3) { ItemBase itemBase = (ItemBase)Activator.CreateInstance(item4); if (itemBase.ParentEquipmentName != null || itemBase.ParentItemName != null) { list4.Add(itemBase); } else if (ValidateItem(itemBase, Items)) { itemBase.Init(((BaseUnityPlugin)this).Config); list3.Add(itemBase.ItemName); } } foreach (ItemBase item5 in list4) { if (list3.Contains(item5.ParentItemName) || list.Contains(item5.ParentEquipmentName)) { item5.Init(((BaseUnityPlugin)this).Config); } } IEnumerable<Type> enumerable4 = from type in Assembly.GetExecutingAssembly().GetTypes() where !type.IsAbstract && type.IsSubclassOf(typeof(EliteEquipmentBase)) select type; foreach (Type item6 in enumerable4) { EliteEquipmentBase eliteEquipmentBase = (EliteEquipmentBase)Activator.CreateInstance(item6); if (ValidateEliteEquipment(eliteEquipmentBase, EliteEquipments)) { eliteEquipmentBase.Init(((BaseUnityPlugin)this).Config); } } IEnumerable<Type> enumerable5 = from type in Assembly.GetExecutingAssembly().GetTypes() where !type.IsAbstract && type.IsSubclassOf(typeof(ControllerBase)) select type; foreach (Type item7 in enumerable5) { ControllerBase controllerBase = (ControllerBase)Activator.CreateInstance(item7); controllerBase.Init(((BaseUnityPlugin)this).Config); } IEnumerable<Type> enumerable6 = from type in Assembly.GetExecutingAssembly().GetTypes() where !type.IsAbstract && type.IsSubclassOf(typeof(InteractableBase)) select type; foreach (Type item8 in enumerable6) { InteractableBase interactableBase = (InteractableBase)Activator.CreateInstance(item8); interactableBase.Init(((BaseUnityPlugin)this).Config); } IEnumerable<Type> enumerable7 = from type in Assembly.GetExecutingAssembly().GetTypes() where !type.IsAbstract && type.IsSubclassOf(typeof(CharacterBase)) select type; foreach (Type item9 in enumerable7) { CharacterBase characterBase = (CharacterBase)Activator.CreateInstance(item9); characterBase.Init(((BaseUnityPlugin)this).Config); } } public bool ValidateArtifact(ArtifactBase artifact, List<ArtifactBase> artifactList) { bool value = ((BaseUnityPlugin)this).Config.Bind<bool>("Artifact: " + artifact.ArtifactName, "Enable Artifact?", true, "Should this artifact appear for selection?").Value; if (value) { artifactList.Add(artifact); } return value; } public bool ValidateItem(ItemBase item, List<ItemBase> itemList) { //IL_0012: Unknown result type (might be due to invalid IL or missing references) //IL_0018: Invalid comparison between Unknown and I4 if (item.IsSkillReplacement) { } bool flag = true; bool flag2 = false; if ((int)item.Tier != 5) { flag = ((BaseUnityPlugin)this).Config.Bind<bool>(item.ConfigCategory, "Enable Item?", true, "Should this item appear in runs?").Value; flag2 = ((BaseUnityPlugin)this).Config.Bind<bool>(item.ConfigCategory, "Blacklist Item from AI Use?", false, "Should the AI not be able to obtain this item?").Value; } if (flag) { itemList.Add(item); if (flag2) { item.AIBlacklisted = true; } } return flag; } public bool ValidateEquipment(EquipmentBase equipment, List<EquipmentBase> equipmentList) { if (((BaseUnityPlugin)this).Config.Bind<bool>(equipment.ConfigCategory, "Enable Equipment?", true, "Should this equipment appear in runs?").Value) { equipmentList.Add(equipment); return true; } return false; } public bool ValidateEliteEquipment(EliteEquipmentBase eliteEquipment, List<EliteEquipmentBase> eliteEquipmentList) { if (((BaseUnityPlugin)this).Config.Bind<bool>("Equipment: " + eliteEquipment.EliteEquipmentName, "Enable Elite Equipment?", true, "Should this elite equipment appear in runs? If disabled, the associated elite will not appear in runs either.").Value) { eliteEquipmentList.Add(eliteEquipment); return true; } return false; } } internal class ModSupport { internal static class BetterUICompat { [MethodImpl(MethodImplOptions.NoInlining | MethodImplOptions.NoOptimization)] internal static void StatsDisplay() { //IL_000d: Unknown result type (might be due to invalid IL or missing references) //IL_0017: Expected O, but got Unknown //IL_0024: Unknown result type (might be due to invalid IL or missing references) //IL_002e: Expected O, but got Unknown //IL_003b: Unknown result type (might be due to invalid IL or missing references) //IL_0045: Expected O, but got Unknown StatsDisplay.AddStatsDisplay("$riskofbulletstorm_accuracy", new DisplayCallback(GetAccuracy)); StatsDisplay.AddStatsDisplay("$riskofbulletstorm_curse", new DisplayCallback(GetCurse)); StatsDisplay.AddStatsDisplay("$riskofbulletstorm_steal", new DisplayCallback(GetStealChance)); } [MethodImpl(MethodImplOptions.NoInlining | MethodImplOptions.NoOptimization)] internal static void ItemTags() { } [MethodImpl(MethodImplOptions.NoInlining | MethodImplOptions.NoOptimization)] internal static void BetterUICompat_RegisterTag() { //IL_0001: Unknown result type (might be due to invalid IL or missing references) //IL_0006: Unknown result type (might be due to invalid IL or missing references) //IL_0010: Expected O, but got Unknown //IL_0010: Expected O, but got Unknown ItemStats.RegisterTag(new ItemStat(), new ItemTag()); } [MethodImpl(MethodImplOptions.NoInlining | MethodImplOptions.NoOptimization)] internal static void BuffsDisplay() { string prefix = "RISKOFBULLETSTORM_BUFF_"; } [MethodImpl(MethodImplOptions.NoInlining | MethodImplOptions.NoOptimization)] internal static void ItemStats() { //IL_002e: Unknown result type (might be due to invalid IL or missing references) //IL_003e: Expected O, but got Unknown //IL_0065: Unknown result type (might be due to invalid IL or missing references) //IL_0079: Expected O, but got Unknown //IL_009b: Unknown result type (might be due to invalid IL or missing references) //IL_00ab: Expected O, but got Unknown //IL_00d2: Unknown result type (might be due to invalid IL or missing references) //IL_00e6: Expected O, but got Unknown //IL_010d: Unknown result type (might be due to invalid IL or missing references) //IL_011d: Expected O, but got Unknown //IL_013f: Unknown result type (might be due to invalid IL or missing references) //IL_0153: Expected O, but got Unknown //IL_017a: Unknown result type (might be due to invalid IL or missing references) //IL_018a: Expected O, but got Unknown //IL_01b1: Unknown result type (might be due to invalid IL or missing references) //IL_01c1: Expected O, but got Unknown //IL_01e8: Unknown result type (might be due to invalid IL or missing references) //IL_01f8: Expected O, but got Unknown //IL_021a: Unknown result type (might be due to invalid IL or missing references) //IL_022a: Expected O, but got Unknown //IL_0251: Unknown result type (might be due to invalid IL or missing references) //IL_0261: Expected O, but got Unknown //IL_0288: Unknown result type (might be due to invalid IL or missing references) //IL_0298: Expected O, but got Unknown //IL_02bf: Unknown result type (might be due to invalid IL or missing references) //IL_02d3: Expected O, but got Unknown //IL_02f5: Unknown result type (might be due to invalid IL or missing references) //IL_0309: Expected O, but got Unknown //IL_032b: Unknown result type (might be due to invalid IL or missing references) //IL_033f: Expected O, but got Unknown //IL_0361: Unknown result type (might be due to invalid IL or missing references) //IL_0371: Expected O, but got Unknown //IL_0398: Unknown result type (might be due to invalid IL or missing references) //IL_03a8: Expected O, but got Unknown //IL_03cf: Unknown result type (might be due to invalid IL or missing references) //IL_03df: Expected O, but got Unknown //IL_0406: Unknown result type (might be due to invalid IL or missing references) //IL_0416: Expected O, but got Unknown //IL_0438: Unknown result type (might be due to invalid IL or missing references) //IL_044c: Expected O, but got Unknown //IL_0475: Unknown result type (might be due to invalid IL or missing references) //IL_0485: Expected O, but got Unknown string text = "RISKOFBULLETSTORM_STAT_"; ItemStats.RegisterStat(ItemBase<Antibody>.instance.ItemDef, text + "ANTIBODY_HEALCHANCE", Antibody.cfgChance / 100f, new StackingFormula(ItemStats.NoStacking), StatFormatter.Chance, (ItemTag)null); ItemStats.RegisterStat(ItemBase<Antibody>.instance.ItemDef, text + "ANTIBODY_HEALAMOUNT", Antibody.cfgMultiplier, Antibody.cfgMultiplierPerStack, new StackingFormula(ItemStats.LinearStacking), StatFormatter.Percent, ItemTag.Healing); ItemStats.RegisterStat(ItemBase<Mustache>.instance.ItemDef, text + "MUSTACHE_DURATION", Mustache.cfgDuration, new StackingFormula(ItemStats.NoStacking), StatFormatter.Seconds, (ItemTag)null); ItemStats.RegisterStat(ItemBase<Mustache>.instance.ItemDef, text + "MUSTACHE_REGEN", Mustache.cfgRegenAmount, Mustache.cfgRegenAmountPerStack, new StackingFormula(ItemStats.LinearStacking), StatFormatter.Regen, ItemTag.Healing); ItemStats.RegisterStat(ItemBase<Scope>.instance.ItemDef, text + "SCOPE_SPREADREDUCTION", Scope.cfgSpreadReduction, Scope.cfgSpreadReductionPerStack, new StackingFormula(ItemStats.LinearStacking), StatFormatter.Percent, (ItemTag)null); ItemStats.RegisterStat(ItemBase<BattleStandard>.instance.ItemDef, text + "BATTLESTANDARD_DAMAGEBONUS", BattleStandard.damage, new StackingFormula(ItemStats.LinearStacking), StatFormatter.Percent, ItemTag.Damage); ItemStats.RegisterStat(ItemBase<CoinCrown>.instance.ItemDef, text + "COINCROWN_MONEYMULTADD", CoinCrown.cashMultiplier, CoinCrown.cashMultiplierPerStack, new StackingFormula(ItemStats.LinearStacking), StatFormatter.Percent, (ItemTag)null); ItemStats.RegisterStat(ItemBase<BloodiedScarf>.instance.ItemDef, text + "BLOODIEDSCARF_DISTANCE", BloodiedScarf.cfgTeleportRange, BloodiedScarf.cfgTeleportRangePerStack, new StackingFormula(ItemStats.LinearStacking), StatFormatter.Range, (ItemTag)null); ItemStats.RegisterStat(ItemBase<BloodiedScarf>.instance.ItemDef, text + "BLOODIEDSCARF_DAMAGEVULNERABILITY", BloodiedScarf.cfgDamageVulnerabilityMultiplier, BloodiedScarf.cfgDamageVulnerabilityMultiplierPerStack, new StackingFormula(ItemStats.LinearStacking), StatFormatter.Percent, (ItemTag)null); ItemStats.RegisterStat(ItemBase<BloodiedScarf>.instance.ItemDef, text + "BLOODIEDSCARF_VULNERABILITYDURATION", BloodiedScarf.cfgDamageVulnerabilityDuration, new StackingFormula(ItemStats.NoStacking), StatFormatter.Seconds, (ItemTag)null); ItemStats.RegisterStat(ItemBase<DodgeRollUtilityReplacement>.instance.ItemDef, text + "DODGEROLLUTILITYREPLACEMENT_DAMAGEVULNERABILITY", DodgeRollUtilityReplacement.cfgDamageVulnerabilityMultiplier, DodgeRollUtilityReplacement.cfgDamageVulnerabilityMultiplierPerStack, new StackingFormula(ItemStats.LinearStacking), StatFormatter.Percent, (ItemTag)null); ItemStats.RegisterStat(ItemBase<DodgeRollUtilityReplacement>.instance.ItemDef, text + "DODGEROLLUTILITYREPLACEMENT_VULNERABILITYDURATION", DodgeRollUtilityReplacement.cfgDamageVulnerabilityDuration, DodgeRollUtilityReplacement.cfgDamageVulnerabilityDurationDecreasePerStack, new StackingFormula(DodgeRollDurationStacking), StatFormatter.Seconds, (ItemTag)null); ItemStats.RegisterStat(ItemBase<RingMiserlyProtection>.instance.ItemDef, text + "RINGMISERLYPROTECTION_HEALTH", RingMiserlyProtection.cfgMaxHealthPctAdded, RingMiserlyProtection.cfgMaxHealthPctAddedStack, new StackingFormula(ItemStats.LinearStacking), StatFormatter.Percent, ItemTag.MaxHealth); ItemStats.RegisterStat(ItemBase<RiskOfBulletstormRewrite.Items.OrangeConsumed>.instance.ItemDef, text + "ORANGECONSUMED_MAXHEALTHADD", Orange.cfgMaxHealthIncrease, new StackingFormula(ItemStats.LinearStacking), StatFormatter.Percent, ItemTag.MaxHealth); ItemStats.RegisterStat(ItemBase<RiskOfBulletstormRewrite.Items.OrangeConsumed>.instance.ItemDef, text + "ORANGECONSUMED_SKILLREDUCTION", Orange.cfgChargeRateReduction, new StackingFormula(ItemStats.HyperbolicStacking), StatFormatter.Percent, ItemTag.SkillCooldown); ItemStats.RegisterStat(ItemBase<CurseTally>.instance.ItemDef, text + "CURSETALLY_CURSE", 1f, new StackingFormula(ItemStats.LinearStacking), StatFormatter.Charges, (ItemTag)null); ItemStats.RegisterStat(ItemBase<SpiceTally>.instance.ItemDef, text + "SPICETALLY_DAMAGE", Spice2.cfgStatDamage, Spice2.cfgStatDamageStack, new StackingFormula(ItemStats.LinearStacking), StatFormatter.Percent, (ItemTag)null); ItemStats.RegisterStat(ItemBase<SpiceTally>.instance.ItemDef, text + "SPICETALLY_ACCURACY", Spice2.cfgStatAccuracy, Spice2.cfgStatAccuracyStack, new StackingFormula(ItemStats.LinearStacking), StatFormatter.Percent, (ItemTag)null); ItemStats.RegisterStat(ItemBase<SpiceTally>.instance.ItemDef, text + "SPICETALLY_ROR2CURSE", Spice2.cfgStatRORCurse, Spice2.cfgStatRORCurseStack, new StackingFormula(ItemStats.LinearStacking), StatFormatter.Charges, (ItemTag)null); ItemStats.RegisterStat(ItemBase<BattleStandardVoid>.instance.ItemDef, text + "BATTLESTANDARDVOID_DAMAGEBONUSPERMINION", BattleStandardVoid.damage, new StackingFormula(ItemStats.LinearStacking), StatFormatter.Percent, ItemTag.Damage); ItemStats.RegisterStat(ItemBase<CoinCrownVoid>.instance.ItemDef, text + "EXTRACASHPERKILL", (float)CoinCrownVoid.cfgCashAdder, (float)CoinCrownVoid.cfgCashAdderStack, new StackingFormula(ItemStats.LinearStacking), StatFormatter.Charges, (ItemTag)null); } } internal static bool betterUILoaded; internal static void CheckForModSupport() { if (Chainloader.PluginInfos.ContainsKey("com.xoxfaby.BetterUI")) { betterUILoaded = true; BetterUICompat.StatsDisplay(); BetterUICompat.ItemStats(); BetterUICompat.BuffsDisplay(); BetterUICompat.ItemTags(); } } private static string GetFloat(ConfigEntry<float> entry) { return GetFloat(entry); } private static string GetFloat(float value) { return value.ToString(); } private static string GetAccuracy(CharacterBody body) { string result = "N/A"; CharacterMaster master = body.master; if (Object.op_Implicit((Object)(object)master)) { ExtraStatsController.RBSExtraStatsController component = ((Component)master).GetComponent<ExtraStatsController.RBSExtraStatsController>(); if (Object.op_Implicit((Object)(object)component)) { return $"{component.idealizedAccuracyStat * 100f:0.##}%"; } } return result; } private static string GetCurse(CharacterBody body) { string result = "N/A"; CharacterMaster master = body.master; if (Object.op_Implicit((Object)(object)master)) { ExtraStatsController.RBSExtraStatsController component = ((Component)master).GetComponent<ExtraStatsController.RBSExtraStatsController>(); if (Object.op_Implicit((Object)(object)component)) { return $"{component.curse:0.##}"; } } return result; } private static string GetStealChance(CharacterBody body) { int count = ItemBase<StolenItemTally>.instance.GetCount(body); string text = (100 / (count + 1)).ToString("0.##"); return text + "%"; } public static float DodgeRollDurationStacking(float value, float extraStac, int stacks) { return DodgeRollUtilityReplacement.GetDuration(stacks); } public static float CustomHyperbolicStacking(float value, float extraStac, int stacks) { return ItemHelpers.GetHyperbolicValue(value, extraStac, stacks); } } public static class GameEndings { public enum ShowCredits { Default, Show, DontShow } public static GameEndingDef gedPastWon; public static GameEndingDef gedPastLose; public static void Init(ConfigFile config) { } public static void SetupGameEndings() { } public static GameEndingDef CreateGameEndingDef(string name, string endingTextToken, SerializableEntityStateType gameOverControllerState, bool isWin, Color backgroundColor, Color foregroundColor, Sprite icon, Material material, uint lunarCoinReward = 20u, ShowCredits showCredits = ShowCredits.Default, GameObject defaultKillerOverride = null) { //IL_0008: Unknown result type (might be due to invalid IL or missing references) //IL_000a: Unknown result type (might be due to invalid IL or missing references) //IL_0027: Unknown result type (might be due to invalid IL or missing references) //IL_0029: Unknown result type (might be due to invalid IL or missing references) //IL_002f: Unknown result type (might be due to invalid IL or missing references) //IL_0030: Unknown result type (might be due to invalid IL or missing references) GameEndingDef val = ScriptableObject.CreateInstance<GameEndingDef>(); val.backgroundColor = backgroundColor; val.cachedName = name; val.defaultKillerOverride = defaultKillerOverride; val.endingTextToken = endingTextToken; val.foregroundColor = foregroundColor; val.gameOverControllerState = gameOverControllerState; val.icon = icon; val.isWin = isWin; val.lunarCoinReward = lunarCoinReward; val.material = material; ((Object)val).name = name; switch (showCredits) { case ShowCredits.Default: val.showCredits = val.isWin; break; case ShowCredits.Show: val.showCredits = true; break; case ShowCredits.DontShow: val.showCredits = false; break; } ContentAddition.AddGameEndingDef(val); return val; } } public static class Tweaks { public enum NotificationMod { disable, bulletstorm, all } public static ConfigEntry<bool> cfgCenterNotifications; public const string category = "Tweaks"; public static void Init(ConfigFile config) { //IL_0039: Unknown result type (might be due to invalid IL or missing references) //IL_0043: Expected O, but got Unknown //IL_004b: Unknown result type (might be due to invalid IL or missing references) //IL_0055: Expected O, but got Unknown //IL_005d: Unknown result type (might be due to invalid IL or missing references) //IL_0067: Expected O, but got Unknown //IL_006f: Unknown result type (might be due to invalid IL or missing references) //IL_0079: Expected O, but got Unknown MechanicStealing.Init(config); cfgCenterNotifications = config.Bind<bool>("Tweaks", "Center Notification Text", false, "If true, then notification text will be centered."); if (cfgCenterNotifications.Value) { CharacterMasterNotificationQueue.PushNotification += new hook_PushNotification(CharacterMasterNotificationQueue_PushNotification); GenericNotification.SetArtifact += new hook_SetArtifact(SetArtifact); GenericNotification.SetEquipment += new hook_SetEquipment(SetEquipment); GenericNotification.SetItem += new hook_SetItem(SetItem); } } private static void AchievementNotificationPanel_SetAchievementDef(orig_SetAchievementDef orig, AchievementNotificationPanel self, AchievementDef achievementDef) { orig.Invoke(self, achievementDef); } private static void CenterTextMesh(GenericNotification genericNotification) { genericNotification.titleText.textMeshPro.alignment = (TextAlignmentOptions)514; genericNotification.descriptionText.textMeshPro.alignment = (TextAlignmentOptions)514; } private static void SetItem(orig_SetItem orig, GenericNotification self, ItemDef itemDef) { orig.Invoke(self, itemDef); CenterTextMesh(self); } private static void SetEquipment(orig_SetEquipment orig, GenericNotification self, EquipmentDef equipmentDef) { orig.Invoke(self, equipmentDef); CenterTextMesh(self); } private static void SetArtifact(orig_SetArtifact orig, GenericNotification self, ArtifactDef artifactDef) { orig.Invoke(self, artifactDef); CenterTextMesh(self); } private static void CharacterMasterNotificationQueue_PushNotification(orig_PushNotification orig, CharacterMasterNotificationQueue self, NotificationInfo notificationInfo, float duration) { orig.Invoke(self, notificationInfo, duration); } } } namespace RiskOfBulletstormRewrite.MarkdownCreation { public class ReadmeCreator { public static bool isEnabled = true; public static bool showWarning = true; private static StringBuilder sb; public static ItemTier[] desiredTierArrangement; public static Dictionary<ItemTier, string> itemTierTokens; public static bool[] desiredEquipmentArrangement; public static void Initialization() { //IL_0008: Unknown result type (might be due to invalid IL or missing references) //IL_0012: Expected O, but got Unknown MainMenuController.Start += new hook_Start(MainMenuController_Start); } private static void MainMenuController_Start(orig_Start orig, MainMenuController self) { orig.Invoke(self); sb = StringBuilderPool.RentStringBuilder(); CreateHeaderSection(); CreateItemSection(); CreateEquipmentSection(); CreateArtifactSection(); Debug.Log((object)sb.ToString()); } private static void CreateArtifactSection() { List<ArtifactBase> list = Main.Artifacts.Where((ArtifactBase equip) => equip.ArtifactDef.nameToken.StartsWith("RISKOFBULLETSTORM_")).ToList(); sb.AppendLine("## Artifacts"); sb.AppendLine("|Icon| Artifact | Desc |"); sb.AppendLine("|:--:|:--:|--|"); foreach (ArtifactBase item in list) { ArtifactDef artifactDef = item.ArtifactDef; string text = ReplaceStyleTagsWithBackticks(Language.GetString(artifactDef.descriptionToken)); sb.AppendLine($"| }_ENABLED.png) | [**{Language.GetString(artifactDef.nameToken)}**]({item.WikiLink}) | {text}"); } } public static void CreateHeaderSection() { sb.AppendLine("# Risk of Bulletstorm"); sb.AppendLine(""); sb.AppendLine("| [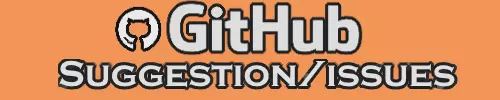](https://github.com/DestroyedClone/RiskOfBulletstorm/issues) | [](https://discord.gg/DpHu3qXMHK) |"); sb.AppendLine("|--|--|"); sb.AppendLine(""); sb.AppendLine("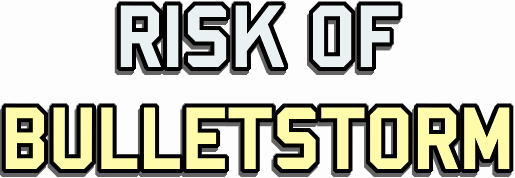"); sb.AppendLine(""); sb.AppendLine("**Risk of Bulletstorm** <sup>(**Risk** of a Rain**storm** of **Bullets**, not Bulletstorm the game!)</sup>, is a content mod that adds items, equipment, and mechanics from the [**Enter The Gungeon**](https://www.dodgeroll.com/gungeon/) property by Dodge Roll Games."); sb.AppendLine(""); if (showWarning) { sb.AppendLine("## Warning"); sb.AppendLine("* Mod's not done so there's missing models/textures/effects/item displays"); sb.AppendLine("* Not tested or setup for multiplayer"); sb.AppendLine("* Not tested for any mod incompats"); } sb.AppendLine(""); if (true) { sb.AppendLine("## Wiki"); sb.AppendLine("There is a rudimentary wiki for this with additional information."); sb.AppendLine("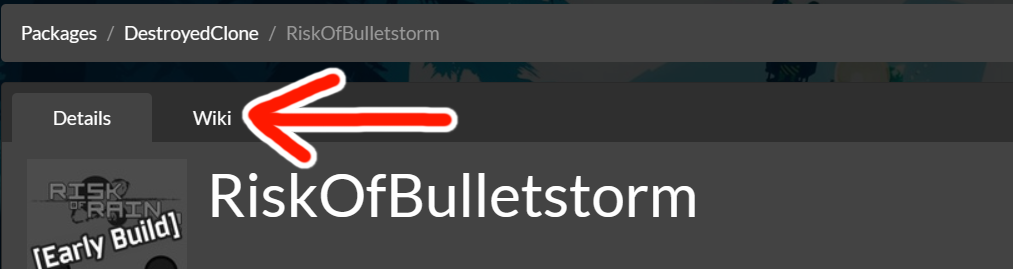"); sb.AppendLine(""); } } public static void CreateItemSection() { //IL_00a9: Unknown result type (might be due to invalid IL or missing references) //IL_00c8: Unknown result type (might be due to invalid IL or missing references) //IL_00ca: Unknown result type (might be due to invalid IL or missing references) //IL_00dc: Unknown result type (might be due to invalid IL or missing references) //IL_00e1: Unknown result type (might be due to invalid IL or missing references) //IL_00e8: Unknown result type (might be due to invalid IL or missing references) List<ItemBase> list = (from item in Main.Items where item.ItemDef.nameToken.StartsWith("RISKOFBULLETSTORM_") where desiredTierArrangement.Contains(item.ItemDef.tier) orderby Array.IndexOf(desiredTierArrangement, item.ItemDef.tier) select item).ToList(); sb.AppendLine("## Items"); sb.AppendLine("|Icon| Item | Pickup |"); sb.AppendLine("|:--:|:--:|--|"); ItemTier val = (ItemTier)5; foreach (ItemBase item in list) { ItemDef itemDef = item.ItemDef; if (val != item.Tier) { val = item.Tier; itemTierTokens.TryGetValue(item.Tier, out var value); sb.AppendLine("| " + value + " |"); } string text = ReplaceStyleTagsWithBackticks(Language.GetString(itemDef.pickupToken)); string text2 = ReplaceStyleTagsWithBackticks(Language.GetString(itemDef.descriptionToken)); sb.AppendLine($"| }.png) | [**{Language.GetString(itemDef.nameToken)}**]({item.WikiLink}) | {text}"); } } public static void CreateEquipmentSection() { List<EquipmentBase> list = (from equip in Main.Equipments where equip.EquipmentDef.nameToken.StartsWith("RISKOFBULLETSTORM_") where equip.EquipmentDef.canDrop orderby Array.IndexOf(desiredEquipmentArrangement, equip.IsLunar) select equip).ToList(); sb.AppendLine("## Equipment"); sb.AppendLine("|Icon| Equipment | Pickup | CD |"); sb.AppendLine("|:--:|:--:|--|--|"); bool flag = false; sb.AppendLine("| Equipment |"); foreach (EquipmentBase item in list) { if (flag != item.IsLunar) { sb.AppendLine("| Lunar Equipment |"); flag = item.IsLunar; } EquipmentDef equipmentDef = item.EquipmentDef; string text = ReplaceStyleTagsWithBackticks(Language.GetString(equipmentDef.pickupToken)); string text2 = ReplaceStyleTagsWithBackticks(Language.GetString(equipmentDef.descriptionToken)); sb.AppendLine($"| }.png) | [**{Language.GetString(equipmentDef.nameToken)}**]({item.WikiLink}) | {text} | {equipmentDef.cooldown}"); } } public static string ReplaceStyleTagsWithBackticks(string input) { string pattern = "<style=[^>]+>(.*?)<\\/style>"; string replacement = "`$1`"; string text = Regex.Replace(input, pattern, replacement); return text.Replace("\n", "<br>"); } public static string GetLangTokenFromToken(string input) { string[] array = new string[5] { "_NAME", "RISKOFBULLETSTORM_", "ITEM_", "EQUIPMENT_", "ARTIFACT_" }; foreach (string oldValue in array) { input = input.Replace(oldValue, ""); } return input; } static ReadmeCreator() { ItemTier[] array = new ItemTier[8]; RuntimeHelpers.InitializeArray(array, (RuntimeFieldHandle)/*OpCode not supported: LdMemberToken*/); desiredTierArrangement = (ItemTier[])(object)array; itemTierTokens = new Dictionary<ItemTier, string> { { (ItemTier)0, "Common" }, { (ItemTier)1, "Uncommon" }, { (ItemTier)2, "Legendary" }, { (ItemTier)4, "Boss" }, { (ItemTier)6, "Void Common" }, { (ItemTier)7, "Void Uncommon" }, { (ItemTier)8, "Void Legendary" }, { (ItemTier)9, "Void Boss" } }; desiredEquipmentArrangement = new bool[2] { false, true }; } } } namespace RiskOfBulletstormRewrite.Utils { internal class AvailabilityManager { public static class EntityStateMachine { public static ResourceAvailability availability = default(ResourceAvailability); } public static void Initialize() { //IL_0008: Unknown result type (might be due to invalid IL or missing references) //IL_0012: Expected O, but got Unknown EntityStateCatalog.Init += new hook_Init(EntityStateCatalog_Init); } private static void EntityStateCatalog_Init(orig_Init orig) { orig.Invoke(); ((ResourceAvailability)(ref EntityStateMachine.availability)).MakeAvailable(); } } public static class Buffs { public static BuffDef MustacheBuff; public static BuffDef MetronomeTrackerBuff; public static BuffDef BloodiedScarfBuff; public static BuffDef AlphaBulletBuff; public static BuffDef DodgeRollBuff; public static BuffDef ArtifactSpeedUpBuff; public static void CreateBuffs() { //IL_0010: Unknown result type (might be due to invalid IL or missing references) //IL_0030: Unknown result type (might be due to invalid IL or missing references) //IL_0050: Unknown result type (might be due to invalid IL or missing references) //IL_0070: Unknown result type (might be due to invalid IL or missing references) //IL_0090: Unknown result type (might be due to invalid IL or missing references) //IL_00b0: Unknown result type (might be due to invalid IL or missing references) MustacheBuff = AddBuff("Power of Commerce", Assets.LoadSprite("BUFF_MUSTACHE"), Color.yellow, isDebuff: false, canStack: true); BloodiedScarfBuff = AddBuff("Vulnerable", Assets.LoadSprite("BUFF_BLOODIEDSCARF"), Color.red, isDebuff: true); AlphaBulletBuff = AddBuff("Alpha Bullet Damage", Assets.LoadSprite("BUFF_ALPHABULLET"), Color.yellow, isDebuff: false, canStack: true); DodgeRollBuff = AddBuff("Dodgeroll Buff", Assets.LoadSprite("BUFF_DODGEROLL"), Color.yellow, isDebuff: true); ArtifactSpeedUpBuff = AddBuff("Speed Up", Assets.LoadSprite("BUFF_SPEEDUPOOC"), Color.blue); MetronomeTrackerBuff = AddBuff("Metronome Stacks (Display)", Assets.LoadSprite("BUFF_METRONOME"), Color.blue, isDebuff: false, canStack: true); } public static BuffDef AddBuff(string name, Sprite iconSprite, Color buffColor, bool isDebuff = false, bool canStack = false) { //IL_0010: Unknown result type (might be due to invalid IL or missing references) //IL_0011: Unknown result type (might be due to invalid IL or missing references) BuffDef val = ScriptableObject.CreateInstance<BuffDef>(); ((Object)val).name = name; val.buffColor = buffColor; val.canStack = canStack; val.isDebuff = isDebuff; val.iconSprite = iconSprite; ContentAddition.AddBuffDef(val); return val; } } internal class HelperDisplays { } internal class ItemDisplays { } internal class EquipmentDisplays { public static ItemDisplayRuleDict Drill(ref GameObject ItemBodyModelPrefab, GameObject EquipmentModel) { //IL_0040: Unknown result type (might be due to invalid IL or missing references) //IL_0049: Unknown result type (might be due to invalid IL or missing references) //IL_0074: Unknown result type (might be due to invalid IL or missing references) //IL_0079: Unknown result type (might be due to invalid IL or missing references) //IL_008f: Unknown result type (might be due to invalid IL or missing references) //IL_0094: Unknown result type (might be due to invalid IL or missing references) //IL_00aa: Unknown result type (might be due to invalid IL or missing references) //IL_00af: Unknown result type (might be due to invalid IL or missing references) //IL_00b4: Unknown result type (might be due to invalid IL or missing references) //IL_00b5: Unknown result type (might be due to invalid IL or missing references) //IL_00ba: Unknown result type (might be due to invalid IL or missing references) //IL_00c0: Expected O, but got Unknown //IL_00d0: Unknown result type (might be due to invalid IL or missing references) //IL_00d9: Unknown result type (might be due to invalid IL or missing references) //IL_0104: Unknown result type (might be due to invalid IL or missing references) //IL_0109: Unknown result type (might be due to invalid IL or missing references) //IL_011f: Unknown result type (might be due to invalid IL or missing references) //IL_0124: Unknown result type (might be due to invalid IL or missing references) //IL_013a: Unknown result type (might be due to invalid IL or missing references) //IL_013f: Unknown result type (might be due to invalid IL or missing references) //IL_0144: Unknown result type (might be due to invalid IL or missing references) //IL_0145: Unknown result type (might be due to invalid IL or missing references) //IL_0160: Unknown result type (might be due to invalid IL or missing references) //IL_0169: Unknown result type (might be due to invalid IL or missing references) //IL_0194: Unknown result type (might be due to invalid IL or missing references) //IL_0199: Unknown result type (might be due to invalid IL or missing references) //IL_01af: Unknown result type (might be due to invalid IL or missing references) //IL_01b4: Unknown result type (might be due to invalid IL or missing references) //IL_01ca: Unknown result type (might be due to invalid IL or missing references) //IL_01cf: Unknown result type (might be due to invalid IL or missing references) //IL_01d4: Unknown result type (might be due to invalid IL or missing references) //IL_01d5: Unknown result type (might be due to invalid IL or missing references) //IL_01f0: Unknown result type (might be due to invalid IL or missing references) //IL_01f9: Unknown result type (might be due to invalid IL or missing references) //IL_0224: Unknown result type (might be due to invalid IL or missing references) //IL_0229: Unknown result type (might be due to invalid IL or missing references) //IL_023f: Unknown result type (might be due to invalid IL or missing references) //IL_0244: Unknown result type (might be due to invalid IL or missing references) //IL_025a: Unknown result type (might be due to invalid IL or missing references) //IL_025f: Unknown result type (might be due to invalid IL or missing references) //IL_0264: Unknown result type (might be due to invalid IL or missing references) //IL_0265: Unknown result type (might be due to invalid IL or missing references) //IL_0280: Unknown result type (might be due to invalid IL or missing references) //IL_0289: Unknown result type (might be due to invalid IL or missing references) //IL_02b4: Unknown result type (might be due to invalid IL or missing references) //IL_02b9: Unknown result type (might be due to invalid IL or missing references) //IL_02cf: Unknown result type (might be due to invalid IL or missing references) //IL_02d4: Unknown result type (might be due to invalid IL or missing references) //IL_02ea: Unknown result type (might be due to invalid IL or missing references) //IL_02ef: Unknown result type (might be due to invalid IL or missing references) //IL_02f4: Unknown result type (might be due to invalid IL or missing references) //IL_02f5: Unknown result type (might be due to invalid IL or missing references) //IL_0310: Unknown result type (might be due to invalid IL or missing references) //IL_0319: Unknown result type (might be due to invalid IL or missing references) //IL_0344: Unknown result type (might be due to invalid IL or missing references) //IL_0349: Unknown result type (might be due to invalid IL or missing references) //IL_035f: Unknown result type (might be due to invalid IL or missing references) //IL_0364: Unknown result type (might be due to invalid IL or missing references) //IL_037a: Unknown result type (might be due to invalid IL or missing references) //IL_037f: Unknown result type (might be due to invalid IL or missing references) //IL_0384: Unknown result type (might be due to invalid IL or missing references) //IL_0385: Unknown result type (might be due to invalid IL or missing references) //IL_03a0: Unknown result type (might be due to invalid IL or missing references) //IL_03a9: Unknown result type (might be due to invalid IL or missing references) //IL_03d4: Unknown result type (might be due to invalid IL or missing references) //IL_03d9: Unknown result type (might be due to invalid IL or missing references) //IL_03ef: Unknown result type (might be due to invalid IL or missing references) //IL_03f4: Unknown result type (might be due to invalid IL or missing references) //IL_040a: Unknown result type (might be due to invalid IL or missing references) //IL_040f: Unknown result type (might be due to invalid IL or missing references) //IL_0414: Unknown result type (might be due to invalid IL or missing references) //IL_0415: Unknown result type (might be due to invalid IL or missing references) //IL_0430: Unknown result type (might be due to invalid IL or missing references) //IL_0439: Unknown result type (might be due to invalid IL or missing references) //IL_0464: Unknown result type (might be due to invalid IL or missing references) //IL_0469: Unknown result type (might be due to invalid IL or missing references) //IL_047f: Unknown result type (might be due to invalid IL or missing references) //IL_0484: Unknown result type (might be due to invalid IL or missing references) //IL_049a: Unknown result type (might be due to invalid IL or missing references) //IL_049f: Unknown result type (might be due to invalid IL or missing references) //IL_04a4: Unknown result type (might be due to invalid IL or missing references) //IL_04a5: Unknown result type (might be due to invalid IL or missing references) //IL_04c0: Unknown result type (might be due to invalid IL or missing references) //IL_04c9: Unknown result type (might be due to invalid IL or missing references) //IL_04f4: Unknown result type (might be due to invalid IL or missing references) //IL_04f9: Unknown result type (might be due to invalid IL or missing references) //IL_050f: Unknown result type (might be due to invalid IL or missing references) //IL_0514: Unknown result type (might be due to invalid IL or missing references) //IL_052a: Unknown result type (might be due to invalid IL or missing references) //IL_052f: Unknown result type (might be due to invalid IL or missing references) //IL_0534: Unknown result type (might be due to invalid IL or missing references) //IL_0535: Unknown result type (might be due to invalid IL or missing references) //IL_0550: Unknown result type (might be due to invalid IL or missing references) //IL_0559: Unknown result type (might be due to invalid IL or missing references) //IL_0584: Unknown result type (might be due to invalid IL or missing references) //IL_0589: Unknown result type (might be due to invalid IL or missing references) //IL_059f: Unknown result type (might be due to invalid IL or missing references) //IL_05a4: Unknown result type (might be due to invalid IL or missing references) //IL_05ba: Unknown result type (might be due to invalid IL or missing references) //IL_05bf: Unknown result type (might be due to invalid IL or missing references) //IL_05c4: Unknown result type (might be due to invalid IL or missing references) //IL_05c5: Unknown result type (might be due to invalid IL or missing references) //IL_05e0: Unknown result type (might be due to invalid IL or missing references) //IL_05e9: Unknown result type (might be due to invalid IL or missing references) //IL_0614: Unknown result type (might be due to invalid IL or missing references) //IL_0619: Unknown result type (might be due to invalid IL or missing references) //IL_062f: Unknown result type (might be due to invalid IL or missing references) //IL_0634: Unknown result type (might be due to invalid IL or missing references) //IL_064a: Unknown result type (might be due to invalid IL or missing references) //IL_064f: Unknown result type (might be due to invalid IL or missing references) //IL_0654: Unknown result type (might be due to invalid IL or missing references) //IL_0655: Unknown result type (might be due to invalid IL or missing references) //IL_0670: Unknown result type (might be due to invalid IL or missing references) //IL_0679: Unknown result type (might be due to invalid IL or missing references) //IL_06a4: Unknown result type (might be due to invalid IL or missing references) //IL_06a9: Unknown result type (might be due to invalid IL or missing references) //IL_06bf: Unknown result type (might be due to invalid IL or missing references) //IL_06c4: Unknown result type (might be due to invalid IL or missing references) //IL_06da: Unknown result type (might be due to invalid IL or missing references) //IL_06df: Unknown result type (might be due to invalid IL or missing references) //IL_06e4: Unknown result type (might be due to invalid IL or missing references) //IL_06e5: Unknown result type (might be due to invalid IL or missing references) //IL_0700: Unknown result type (might be due to invalid IL or missing references) //IL_0709: Unknown result type (might be due to invalid IL or missing references) //IL_0734: Unknown result type (might be due to invalid IL or missing references) //IL_0739: Unknown result type (might be due to invalid IL or missing references) //IL_074f: Unknown result type (might be due to invalid IL or missing references) //IL_0754: Unknown result type (might be due to invalid IL or missing references) //IL_076a: Unknown result type (might be due to invalid IL or missing references) //IL_076f: Unknown result type (might be due to invalid IL or missing references) //IL_0774: Unknown result type (might be due to invalid IL or missing references) //IL_0775: Unknown result type (might be due to invalid IL or missing references) //IL_0790: Unknown result type (might be due to invalid IL or missing references) //IL_0799: Unknown result type (might be due to invalid IL or missing references) //IL_07c4: Unknown result type (might be due to invalid IL or missing references) //IL_07c9: Unknown result type (might be due to invalid IL or missing references) //IL_07df: Unknown result type (might be due to invalid IL or missing references) //IL_07e4: Unknown result type (might be due to invalid IL or missing references) //IL_07fa: Unknown result type (might be due to invalid IL or missing references) //IL_07ff: Unknown result type (might be due to invalid IL or missing references) //IL_0804: Unknown result type (might be due to invalid IL or missing references) //IL_0805: Unknown result type (might be due to invalid IL or missing references) //IL_0820: Unknown result type (might be due to invalid IL or missing references) //IL_0829: Unknown result type (might be due to invalid IL or missing references) //IL_0854: Unknown result type (might be due to invalid IL or missing references) //IL_0859: Unknown result type (might be due to invalid IL or missing references) //IL_086f: Unknown result type (might be due to invalid IL or missing references) //IL_0874: Unknown result type (might be due to invalid IL or missing references) //IL_088a: Unknown result type (might be due to invalid IL or missing references) //IL_088f: Unknown result type (might be due to invalid IL or missing references) //IL_0894: Unknown result type (might be due to invalid IL or missing references) //IL_0895: Unknown result type (might be due to invalid IL or missing references) //IL_08b0: Unknown result type (might be due to invalid IL or missing references) //IL_08b9: Unknown result type (might be due to invalid IL or missing references) //IL_08e4: Unknown result type (might be due to invalid IL or missing references) //IL_08e9: Unknown result type (might be due to invalid IL or missing references) //IL_08ff: Unknown result type (might be due to invalid IL or missing references) //IL_0904: Unknown result type (might be due to invalid IL or missing references) //IL_091a: Unknown result type (might be due to invalid IL or missing references) //IL_091f: Unknown result type (might be due to invalid IL or missing references) //IL_0924: Unknown result type (might be due to invalid IL or missing references) //IL_0925: Unknown result type (might be due to invalid IL or missing references) //IL_0940: Unknown result type (might be due to invalid IL or missing references) //IL_0949: Unknown result type (might be due to invalid IL or missing references) //IL_0974: Unknown result type (might be due to invalid IL or missing references) //IL_0979: Unknown result type (might be due to invalid IL or missing references) //IL_098f: Unknown result type (might be due to invalid IL or missing references) //IL_0994: Unknown result type (might be due to invalid IL or missing references) //IL_09aa: Unknown result type (might be due to invalid IL or missing references) //IL_09af: Unknown result type (might be due to invalid IL or missing references) //IL_09b4: Unknown result type (might be due to invalid IL or missing references) //IL_09b5: Unknown result type (might be due to invalid IL or missing references) //IL_09d0: Unknown result type (might be due to invalid IL or missing references) //IL_09d9: Unknown result type (might be due to invalid IL or missing references) //IL_0a04: Unknown result type (might be due to invalid IL or missing references) //IL_0a09: Unknown result type (might be due to invalid IL or missing references) //IL_0a1f: Unknown result type (might be due to invalid IL or missing references) //IL_0a24: Unknown result type (might be due to invalid IL or missing references) //IL_0a2b: Unknown result type (might be due to invalid IL or missing references) //IL_0a31: Unknown result type (might be due to invalid IL or missing references) //IL_0a36: Unknown result type (might be due to invalid IL or missing references) //IL_0a3b: Unknown result type (might be due to invalid IL or missing references) //IL_0a3c: Unknown result type (might be due to invalid IL or missing references) //IL_0a57: Unknown result type (might be due to invalid IL or missing references) //IL_0a60: Unknown result type (might be due to invalid IL or missing references) //IL_0a8b: Unknown result type (might be due to invalid IL or missing references) //IL_0a90: Unknown result type (might be due to invalid IL or missing references) //IL_0aa6: Unknown result type (might be due to invalid IL or missing references) //IL_0aab: Unknown result type (might be due to invalid IL or missing references) //IL_0ab2: Unknown result type (might be due to invalid IL or missing references) //IL_0ab8: Unknown result type (might be due to invalid IL or missing references) //IL_0abd: Unknown result type (might be due to invalid IL or missing references) //IL_0ac2: Unknown result type (might be due to invalid IL or missing references) //IL_0ac3: Unknown result type (might be due to invalid IL or missing references) //IL_0ade: Unknown result type (might be due to invalid IL or missing references) //IL_0ae7: Unknown result type (might be due to invalid IL or missing references) //IL_0b12: Unknown result type (might be due to invalid IL or missing references) //IL_0b17: Unknown result type (might be due to invalid IL or missing references) //IL_0b2d: Unknown result type (might be due to invalid IL or missing references) //IL_0b32: Unknown result type (might be due to invalid IL or missing references) //IL_0b48: Unknown result type (might be due to invalid IL or missing references) //IL_0b4d: Unknown result type (might be due to invalid IL or missing references) //IL_0b52: Unknown result type (might be due to invalid IL or missing references) //IL_0b53: Unknown result type (might be due to invalid IL or missing references) //IL_0b6e: Unknown result type (might be due to invalid IL or missing references) //IL_0b77: Unknown result type (might be due to invalid IL or missing references) //IL_0ba2: Unknown result type (might be due to invalid IL or missing references) //IL_0ba7: Unknown result type (might be due to invalid IL or missing references) //IL_0bbd: Unknown result type (might be due to invalid IL or missing references) //IL_0bc2: Unknown result type (might be due to invalid IL or missing references) //IL_0bd8: Unknown result type (might be due to invalid IL or missing references) //IL_0bdd: Unknown result type (might be due to invalid IL or missing references) //IL_0be2: Unknown result type (might be due to invalid IL or missing references) //IL_0be3: Unknown result type (might be due to invalid IL or missing references) //IL_0bfe: Unknown result type (might be due to invalid IL or missing references) //IL_0c07: Unknown result type (might be due to invalid IL or missing references) //IL_0c32: Unknown result type (might be due to invalid IL or missing references) //IL_0c37: Unknown result type (might be due to invalid IL or missing references) //IL_0c4d: Unknown result type (might be due to invalid IL or missing references) //IL_0c52: Unknown result type (might be due to invalid IL or missing references) //IL_0c68: Unknown result type (might be due to invalid IL or missing references) //IL_0c6d: Unknown result type (might be due to invalid IL or missing references) //IL_0c72: Unknown result type (might be due to invalid IL or missing references) //IL_0c73: Unknown result type (might be due to invalid IL or missing references) //IL_0c8e: Unknown result type (might be due to invalid IL or missing references) //IL_0c97: Unknown result type (might be due to invalid IL or missing references) //IL_0cc2: Unknown result type (might be due to invalid IL or missing references) //IL_0cc7: Unknown result type (might be due to invalid IL or missing references) //IL_0cdd: Unknown result type (might be due to invalid IL or missing references) //IL_0ce2: Unknown result type (might be due to invalid IL or missing references) //IL_0cf8: Unknown result type (might be due to invalid IL or missing references) //IL_0cfd: Unknown result type (might be due to invalid IL or missing references) //IL_0d02: Unknown result type (might be due to invalid IL or missing references) //IL_0d03: Unknown result type (might be due to invalid IL or missing references) //IL_0d1e: Unknown result type (might be due to invalid IL or missing references) //IL_0d27: Unknown result type (might be due to invalid IL or missing references) //IL_0d52: Unknown result type (might be due to invalid IL or missing references) //IL_0d57: Unknown result type (might be due to invalid IL or missing references) //IL_0d6d: Unknown result type (might be due to invalid IL or missing references) //IL_0d72: Unknown result type (might be due to invalid IL or missing references) //IL_0d88: Unknown result type (might be due to invalid IL or missing references) //IL_0d8d: Unknown result type (might be due to invalid IL or missing references) //IL_0d92: Unknown result type (might be due to invalid IL or missing references) //IL_0d93: Unknown result type (might be due to invalid IL or missing references) //IL_0dae: Unknown result type (might be due to invalid IL or missing references) //IL_0db7: Unknown result type (might be due to invalid IL or missing references) //IL_0de2: Unknown result type (might be due to invalid IL or missing references) //IL_0de7: Unknown result type (might be due to invalid IL or missing references) //IL_0dfd: Unknown result type (might be due to invalid IL or missing references) //IL_0e02: Unknown result type (might be due to invalid IL or missing references) //IL_0e18: Unknown result type (might be due to invalid IL or missing references) //IL_0e1d: Unknown result type (might be due to invalid IL or missing references) //IL_0e22: Unknown result type (might be due to invalid IL or missing references) //IL_0e23: Unknown result type (might be due to invalid IL or missing references) ItemBodyModelPrefab = EquipmentModel; ItemBodyModelPrefab.AddComponent<ItemDisplay>(); ItemBodyModelPrefab.GetComponent<ItemDisplay>().rendererInfos = ItemHelpers.ItemDisplaySetup(ItemBodyModelPrefab); Vector3 val = default(Vector3); ((Vector3)(ref val))..ctor(0.05f, 0.05f, 0.05f); ItemDisplayRuleDict val2 = new ItemDisplayRuleDict((ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Head", localPos = new Vector3(0f, 0f, 0f), localAngles = new Vector3(0f, 0f, 0f), localScale = new Vector3(0f, 0f, 0f) } }); val2.Add("mdlCommandoDualies", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "ThighL", localPos = new Vector3(0.12093f, 0.00085f, 0.00428f), localAngles = new Vector3(0f, 0f, 0f), localScale = new Vector3(0.05f, 0.05f, 0.05f) } }); val2.Add("mdlHuntress", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "ThighR", localPos = new Vector3(-0.10149f, 0.07143f, 0.12604f), localAngles = new Vector3(346.3554f, 35.91308f, 4.99845f), localScale = new Vector3(0.05f, 0.05f, 0.05f) } }); val2.Add("mdlToolbot", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "LeftArmController", localPos = new Vector3(-0.08166f, 1.84028f, -0.71204f), localAngles = new Vector3(49.66273f, 100.7644f, 357.8215f), localScale = new Vector3(0.43029f, 0.43029f, 0.43029f) } }); val2.Add("mdlEngi", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Pelvis", localPos = new Vector3(0.13968f, -0.07825f, -0.19087f), localAngles = new Vector3(1.41354f, 224.5392f, 192.9316f), localScale = new Vector3(0.05f, 0.05f, 0.05f) } }); val2.Add("mdlEngiTurret", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Base", localPos = new Vector3(0f, 1.3612f, 0.25f), localAngles = new Vector3(-2E-05f, 242.8639f, 359.94f), localScale = new Vector3(0.25f, 0.25f, 0.25f) } }); val2.Add("mdlMage", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Pelvis", localPos = new Vector3(-0.1777f, 0.04814f, -0.02098f), localAngles = new Vector3(12.31357f, 0f, 21.55264f), localScale = new Vector3(0.05f, 0.05f, 0.05f) } }); val2.Add("mdlMerc", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Pelvis", localPos = new Vector3(-0.15142f, 0.06104f, -0.15338f), localAngles = new Vector3(4.11953f, 125.5684f, 162.9413f), localScale = new Vector3(0.05f, 0.05f, 0.05f) } }); val2.Add("mdlTreebot", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "HeadBase", localPos = new Vector3(0f, -0.55314f, -0.39199f), localAngles = new Vector3(344.4032f, 180f, 180f), localScale = new Vector3(0.1341f, 0.1341f, 0.1341f) } }); val2.Add("mdlLoader", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Pelvis", localPos = new Vector3(-0.15742f, -0.07828f, -0.11081f), localAngles = new Vector3(6.28547f, 301.9267f, 173.0495f), localScale = new Vector3(0.05f, 0.05f, 0.05f) } }); val2.Add("mdlCroco", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Pelvis", localPos = new Vector3(-1.30804f, 0.20354f, -1.05956f), localAngles = new Vector3(356.3902f, 131.4558f, 159.2798f), localScale = new Vector3(0.33784f, 0.33784f, 0.33784f) } }); val2.Add("mdlCaptain", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Pelvis", localPos = new Vector3(-0.20488f, -0.02376f, -0.12087f), localAngles = new Vector3(357.5009f, 298.8111f, 182.4467f), localScale = new Vector3(0.07467f, 0.07467f, 0.07467f) } }); val2.Add("mdlBrother", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Pelvis", localPos = new Vector3(-0.20832f, -0.13072f, 0.04832f), localAngles = new Vector3(359.1603f, 20.94127f, 196.4378f), localScale = new Vector3(0.07689f, 0.07689f, 0.07689f) } }); val2.Add("mdlBandit", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Pelvis", localPos = new Vector3(-0.09392f, -0.05173f, -0.16504f), localAngles = new Vector3(4.81421f, 295.4022f, 182.498f), localScale = new Vector3(0.05f, 0.05f, 0.05f) } }); val2.Add("mdlBandit2", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Pelvis", localPos = new Vector3(-0.07271f, -0.03641f, -0.18515f), localAngles = new Vector3(8.9437f, 311.8177f, 190.7293f), localScale = new Vector3(0.05f, 0.05f, 0.05f) } }); val2.Add("mdlVoidSurvivor", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Pelvis", localPos = new Vector3(0.08533f, 0.02237f, 0.1406f), localAngles = new Vector3(3.89962f, 159.6285f, 190.3786f), localScale = new Vector3(0.03423f, 0.03423f, 0.03423f) } }); val2.Add("mdlRailGunner", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Pelvis", localPos = new Vector3(0.16028f, 0.03272f, -0.0624f), localAngles = new Vector3(359.8894f, 235.8844f, 191.4629f), localScale = new Vector3(0.06497f, 0.06497f, 0.06497f) } }); val2.Add("mdlClayBruiser", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Head", localPos = new Vector3(0f, 0.1f, 0.4f), localAngles = new Vector3(0f, 0f, 0f), localScale = val * 2f } }); val2.Add("mdlHAND", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Head", localPos = new Vector3(0f, 0f, 2.4f), localAngles = new Vector3(0f, 0f, 0f), localScale = val * 10f } }); val2.Add("mdlScav", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Head", localPos = new Vector3(0f, 4.8685f, 0.0438f), localAngles = new Vector3(288.4044f, 180f, 180f), localScale = new Vector3(1f, 1f, 1f) } }); val2.Add("mdlBeetle", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Head", localPos = new Vector3(0.0013f, 0.1559f, -0.2403f), localAngles = new Vector3(0f, 0f, 0f), localScale = new Vector3(0.1f, 0.1f, 0.1f) } }); val2.Add("mdlLemurian", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Head", localPos = new Vector3(-0.1594f, 3.6456f, 0.0645f), localAngles = new Vector3(279.4401f, 195.4454f, 161.8801f), localScale = new Vector3(0.4099f, 0.4099f, 0.4099f) } }); val2.Add("mdlLunarGolem", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "MuzzleLB", localPos = new Vector3(-1.6752f, -0.2f, -0.468f), localAngles = new Vector3(2.6768f, 179.4175f, 179.4478f), localScale = new Vector3(0.1793f, 0.1793f, 0.1793f) } }); val2.Add("mdlNullifier", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Muzzle", localPos = new Vector3(0.0002f, -0.189f, 1.9457f), localAngles = new Vector3(24.2706f, 0.0024f, 0.024f), localScale = new Vector3(0.2908f, 0.2908f, 0.2908f) } }); val2.Add("mdlGravekeeper", (ItemDisplayRule[])(object)new ItemDisplayRule[1] { new ItemDisplayRule { ruleType = (ItemDisplayRuleType)0, followerPrefab = ItemBodyModelPrefab, childName = "Mask", localPos = new Vector3(0f, 0.0344f, -1.6055f), localAngles = new Vector3(88.6293f, 0f, 0f), localScale = new Vector3(0.425f, 0.425f, 0.425f) } }); return val2; } } internal class EliteDisplays { } internal class ItemHelpers { public static ExpansionDef sotvExpansionDef; public static RendererInfo[] ItemDisplaySetup(GameObject obj, bool debugmode = false) { //IL_0085: Unknown result type (might be due to invalid IL or missing references) //IL_00cf: Unknown result type (might be due to invalid IL or missing references) //IL_00dc: Unknown result type (might be due to invalid IL or missing references) //IL_00de: Unknown result type (might be due to invalid IL or missing references) List<Renderer> list = new List<Renderer>(); MeshRenderer[] componentsInChildren = obj.GetComponentsInChildren<MeshRenderer>(); if (componentsInChildren.Length != 0) { list.AddRange((IEnumerable<Renderer>)(object)componentsInChildren); } SkinnedMeshRenderer[] componentsInChildren2 = obj.GetComponentsInChildren<SkinnedMeshRenderer>(); if (componentsInChildren2.Length != 0) { list.AddRange((IEnumerable<Renderer>)(object)componentsInChildren2); } RendererInfo[] array = (RendererInfo[])(object)new RendererInfo[list.Count]; for (int i = 0; i < list.Count; i++) { if (debugmode) { MaterialControllerComponents.HGControllerFinder hGControllerFinder = ((Component)list[i]).gameObject.AddComponent<MaterialControllerComponents.HGControllerFinder>(); hGControllerFinder.Renderer = list[i]; } array[i] = new RendererInfo { defaultMaterial = ((list[i] is SkinnedMeshRenderer) ? list[i].sharedMaterial : list[i].material), renderer = list[i], defaultShadowCastingMode = (ShadowCastingMode)1, ignoreOverlays = false }; } return array; } public static string Pct(float value) { return $"{100f * value}%"; } public static void SimulatePickup(CharacterMaster characterMaster, ItemDef itemDef, int amount = 1, bool showNotif = true) { //IL_0009: Unknown result type (might be due to invalid IL or missing references) //IL_000e: Unknown result type (might be due to invalid IL or missing references) //IL_0013: Unknown result type (might be due to invalid IL or missing references) //IL_0014: Unknown result type (might be due to invalid IL or missing references) //IL_0015: Unknown result type (might be due to invalid IL or missing references) //IL_003f: Unknown result type (might be due to invalid IL or missing references) Inventory inventory = characterMaster.inventory; PickupIndex val = PickupCatalog.FindPickupIndex(itemDef.itemIndex); if (val > PickupIndex.none) { Util.PlaySound("Play_UI_item_pickup", characterMaster.GetBodyObject()); inventory.GiveItem(itemDef, amount); GenericPickupController.SendPickupMessage(characterMaster, val); if (!showNotif) { } } } public static float GetHyperbolicValue(float baseAmount, float stackAmount) { return 1f - 1f / (1f + baseAmount * stackAmount); } public static float GetHyperbolicValue(float baseAmount, float stackAmount, int itemCount) { if (itemCount <= 1) { return baseAmount * (float)itemCount; } float num = 1f + baseAmount * (stackAmount * (float)(itemCount - 1)); return 1f - 1f / num; } public static int GetEquipmentCountForTeam(TeamIndex teamIndex, EquipmentIndex equipmentIndex, bool requiresAlive, bool requiresConnected = true) { //IL_0020: Unknown result type (might be due to invalid IL or missing references) //IL_0025: Unknown result type (might be due to invalid IL or missing references) int result = 0; ReadOnlyCollection<CharacterMaster> readOnlyInstancesList = CharacterMaster.readOnlyInstancesList; int i = 0; for (int count = readOnlyInstancesList.Count; i < count; i++) { CharacterMaster val = readOnlyInstancesList[i]; if (val.teamIndex != teamIndex || (requiresAlive && !val.hasBody) || !requiresConnected || !Object.op_Implicit((Object)(object)val.playerCharacterMasterController) || val.playerCharacterMasterController.isConnected) { } } return result; } public static bool GetAtLeastOneEquipmentForTeam(TeamIndex teamIndex, EquipmentIndex equipmentIndex, bool requiresAlive, bool requiresConnected = true) { //IL_001c: Unknown result type (might be due to invalid IL or missing references) //IL_0021: Unknown result type (might be due to invalid IL or missing references) //IL_006a: Unknown result type (might be due to invalid IL or missing references) //IL_006f: Unknown result type (might be due to invalid IL or missing references) ReadOnlyCollection<CharacterMaster> readOnlyInstancesList = CharacterMaster.readOnlyInstancesList; int i = 0; for (int count = readOnlyInstancesList.Count; i < count; i++) { CharacterMaster val = readOnlyInstancesList[i]; if (val.teamIndex == teamIndex && (!requiresAlive || val.hasBody) && (!requiresConnected || !Object.op_Implicit((Object)(object)val.playerCharacterMasterController) || val.playerCharacterMasterController.isConnected) && Object.op_Implicit((Object)(object)val.inventory) && val.inventory.currentEquipmentIndex == equipmentIndex) { return true; } } return false; } public static void FireSquidShot(CharacterBody ownerBody, Vector3 origin, float damageCoefficient, float procCoefficient = 1f) { //IL_0001: Unknown result type (might be due to invalid IL or missing references) //IL_0006: Unknown result type (might be due to invalid IL or missing references) //IL_000d: Unknown result type (might be due to invalid IL or missing references) //IL_0018: Unknown result type (might be due to invalid IL or missing references) //IL_0019: Unknown result type (might be due to invalid IL or missing references) //IL_001a: Unknown result type (might be due to invalid IL or missing references) //IL_001f: Unknown result type (might be due to invalid IL or missing references) //IL_0020: Unknown result type (might be due to invalid IL or missing references) //IL_0025: Unknown result type (might be due to invalid IL or missing references) //IL_002a: Unknown result type (might be due to invalid IL or missing references) //IL_002c: Unknown result type (might be due to invalid IL or missing references) //IL_0031: Unknown result type (might be due to invalid IL or missing references) //IL_0032: Unknown result type (might be due to invalid IL or missing references) //IL_0037: Unknown result type (might be due to invalid IL or missing references) //IL_003c: Unknown result type (might be due to invalid IL or missing references) //IL_0047: Unknown result type (might be due to invalid IL or missing references) //IL_0053: Unknown result type (might be due to invalid IL or missing references) //IL_005b: Expected O, but got Unknown //IL_005b: Unknown result type (might be due to invalid IL or missing references) //IL_0061: Invalid comparison between Unknown and I4 //IL_0074: Unknown result type (might be due to invalid IL or missing references) //IL_00a1: Unknown result type (might be due to invalid IL or missing references) //IL_00a6: Unknown result type (might be due to invalid IL or missing references) //IL_00b4: Unknown result type (might be due to invalid IL or missing references) //IL_00cb: Unknown result type (might be due to invalid IL or missing references) //IL_00d2: Unknown result type (might be due to invalid IL or missing references) //IL_00d7: Unknown result type (might be due to invalid IL or missing references) //IL_00dc: Unknown result type (might be due to invalid IL or missing references) //IL_00e8: Unknown result type (might be due to invalid IL or missing references) //IL_00f1: Expected O, but got Unknown //IL_0104: Unknown result type (might be due to invalid IL or missing references) //IL_0105: Unknown result type (might be due to invalid IL or missing references) BullseyeSearch val = new BullseyeSearch { viewer = ownerBody, maxDistanceFilter = float.PositiveInfinity, searchOrigin = origin, searchDirection = Vector3.up, sortMode = (SortMode)1, teamMaskFilter = TeamMask.allButNeutral, minDistanceFilter = 0f, maxAngleFilter = 180f, filterByLoS = true }; if ((int)FriendlyFireManager.friendlyFireMode > 0) { ((TeamMask)(ref val.teamMaskFilter)).RemoveTeam(ownerBody.teamComponent.teamIndex); } val.RefreshCandidates(); HurtBox val2 = val.GetResults().FirstOrDefault(); if (Object.op_Implicit((Object)(object)val2)) { SquidOrb val3 = new SquidOrb { damageValue = ownerBody.damage * damageCoefficient, isCrit = Util.CheckRoll(ownerBody.crit, ownerBody.master), teamIndex = TeamComponent.GetObjectTeam(((Component)ownerBody).gameObject), attacker = ((Component)ownerBody).gameObject, procCoefficient = procCoefficient }; HurtBox val4 = val2; if (Object.op_Implicit((Object)(object)val4)) { ((Orb)val3).origin = origin; ((Orb)val3).target = val4; OrbManager.instance.AddOrb((Orb)(object)val3); } } } public static T Load<T>(string assetPath) { //IL_0002: Unknown result type (might be due to invalid IL or missing references) //IL_0007: Unknown result type (might be due to invalid IL or missing references) return Addressables.LoadAssetAsync<T>((object)assetPath).WaitForCompletion(); } public static ExpansionDef GetSOTVExpansionDef() { //IL_0012: Unknown result type (might be due to invalid IL or missing references) if (!Object.op_Implicit((Object)(object)sotvExpansionDef)) { sotvExpansionDef = ((IEnumerable<ExpansionDef>)(object)ExpansionCatalog.expansionDefs).FirstOrDefault((Func<ExpansionDef, bool>)((ExpansionDef def) => def.nameToken == "DLC1_NAME")); } return sotvExpansionDef; } } } namespace RiskOfBulletstormRewrite.Utils.Components { public class MaterialControllerComponents { public class HGControllerFinder : MonoBehaviour { public Renderer Renderer; public Material Material; public void Start() { if (Object.op_Implicit((Object)(object)Renderer)) { Material = Renderer.materials[0]; if (Object.op_Implicit((Object)(object)Material)) { switch (((Object)Material.shader).name) { case "Hopoo Games/Deferred/Standard": { HGStandardController hGStandardController = ((Component)this).gameObject.AddComponent<HGStandardController>(); hGStandardController.Material = Material; hGStandardController.Renderer = Renderer; break; } case "Hopoo Games/FX/Cloud Remap": { HGCloudRemapController hGCloudRemapController = ((Component)this).gameObject.AddComponent<HGCloudRemapController>(); hGCloudRemapController.Material = Material; hGCloudRemapController.Renderer = Renderer; break; } case "Hopoo Games/FX/Cloud Intersection Remap": { HGIntersectionController hGIntersectionController = ((Component)this).gameObject.AddComponent<HGIntersectionController>(); hGIntersectionController.Material = Material; hGIntersectionController.Renderer = Renderer; break; } } } } Object.Destroy((Object)(object)this); } } public class HGStandardController : MonoBehaviour { public enum _RampInfoEnum { TwoTone = 0, SmoothedTwoTone = 1, Unlitish = 3, Subsurface = 4, Grass = 5 } public enum _DecalLayerEnum { Default, Environment, Character, Misc } public enum _CullEnum { Off, Front, Back } public enum _PrintDirectionEnum { BottomUp = 0, TopDown = 1, BackToFront = 3 } public Material Material; public Renderer Renderer; public string MaterialName; public bool _EnableCutout; public Color _Color; public Texture _MainTex; public Vector2 _MainTexScale; public Vector2 _MainTexOffset; [Range(0f, 5f)] public float _NormalStrength; public Texture _NormalTex; public Vector2 _NormalTexScale; public Vector2 _NormalTexOffset; public Color _EmColor; public Texture _EmTex; [Range(0f, 10f)] public float _EmPower; [Range(0f, 1f)] public float _Smoothness; public bool _IgnoreDiffuseAlphaForSpeculars; public _RampInfoEnum _RampChoice; public _DecalLayerEnum _DecalLayer; [Range(0f, 1f)] public float _SpecularStrength; [Range(0.1f, 20f)] public float _SpecularExponent; public _CullEnum _Cull_Mode; public bool _EnableDither; [Range(0f, 1f)] public float _FadeBias; public bool _EnableFresnelEmission; public Texture _FresnelRamp; [Range(0.1f, 20f)] public float _FresnelPower; public Texture _FresnelMask; [Range(0f, 20f)] public float _FresnelBoost; public bool _EnablePrinting; [Range(-25f, 25f)] public float _SliceHeight; [Range(0f, 10f)] public float _PrintBandHeight; [Range(0f, 1f)] public float _PrintAlphaDepth; public Texture _PrintAlphaTexture; public Vector2 _PrintAlphaTextureScale; public Vector2 _PrintAlphaTextureOffset; [Range(0f, 10f)] public float _PrintColorBoost; [Range(0f, 4f)] public float _PrintAlphaBias; [Range(0f, 1f)] public float _PrintEmissionToAlbedoLerp; public _PrintDirectionEnum _PrintDirection; public Texture _PrintRamp; [Range(-10f, 10f)] public float _EliteBrightnessMin; [Range(-10f, 10f)] public float _EliteBrightnessMax; public bool _EnableSplatmap; public bool _UseVertexColorsInstead; [Range(0f, 1f)] public float _BlendDepth; public Texture _SplatmapTex; public Vector2 _SplatmapTexScale; public Vector2 _SplatmapTexOffset; [Range(0f, 20f)] public float _SplatmapTileScale; public Texture _GreenChannelTex; public Texture _GreenChannelNormalTex; [Range(0f, 1f)] public float _GreenChannelSmoothness; [Range(-2f, 5f)] public float _GreenChannelBias; public Texture _BlueChannelTex; public Texture _BlueChannelNormalTex; [Range(0f, 1f)] public float _BlueChannelSmoothness; [Range(-2f, 5f)] public float _BlueChannelBias; public bool _EnableFlowmap; public Texture _FlowTexture; public Texture _FlowHeightmap; public Vector2 _FlowHeightmapScale; public Vector2 _FlowHeightmapOffset; public Texture _FlowHeightRamp; public Vector2 _FlowHeightRampScale; public Vector2 _FlowHeightRampOffset; [Range(-1f, 1f)] public float _FlowHeightBias; [Range(0.1f, 20f)] public float _FlowHeightPower; [Range(0.1f, 20f)] public float _FlowEmissionStrength; [Range(0f, 15f)] public float _FlowSpeed; [Range(0f, 5f)] public float _MaskFlowStrength; [Range(0f, 5f)] public float _NormalFlowStrength; [Range(0f, 10f)] public float _FlowTextureScaleFactor; public bool _EnableLimbRemoval; public void Start() { GrabMaterialValues(); } public void GrabMaterialValues() { //IL_0036: Unknown result type (might be due to invalid IL or missing references) //IL_003b: Unknown result type (might be due to invalid IL or missing references) //IL_0062: Unknown result type (might be due to invalid IL or missing references) //IL_0067: Unknown result type (might be due to invalid IL or missing references) //IL_0078: Unknown result type (might be due to invalid IL or missing references) //IL_007d: Unknown result type (might be due to invalid IL or missing references) //IL_00ba: Unknown result type (might be due to invalid IL or missing references) //IL_00bf: Unknown result type (might be due to invalid IL or missing references) //IL_00d0: Unknown result type (might be due to invalid IL or missing references) //IL_00d5: Unknown result type (might be due to invalid IL or missing references) //IL_00e6: Unknown result type (might be due to invalid IL or missing references) //IL_00eb: Unknown result type (might be due to invalid IL or missing references) //IL_02cd: Unknown result type (might be due to invalid IL or missing references) //IL_02d2: Unknown result type (might be due to invalid IL or missing references) //IL_02e3: Unknown result type (might be due to invalid IL or missing references) //IL_02e8: Unknown result type (might be due to invalid IL or missing references) //IL_03ec: Unknown result type (might be due to invalid IL or missing references) //IL_03f1: Unknown result type (might be due to invalid IL or missing references) //IL_0402: Unknown result type (might be due to invalid IL or missing references) //IL_0407: Unknown result type (might be due to invalid IL or missing references) //IL_0520: Unknown result type (might be due to invalid IL or missing references) //IL_0525: Unknown result type (might be due to invalid IL or missing references) //IL_0536: Unknown result type (might be due to invalid IL or missing references) //IL_053b: Unknown result type (might be due to invalid IL or missing references) //IL_0562: Unknown result type (might be due to invalid IL or missing references) //IL_0567: Unknown result type (might be due to invalid IL or missing references) //IL_0578: Unknown result type (might be due to invalid IL or missing references) //IL_057d: Unknown result type (might be due to invalid IL or missing references) if (Object.op_Implicit((Object)(object)Material)) { _EnableCutout = Material.IsKeywordEnabled("CUTOUT"); _Color = Material.GetColor("_Color"); _MainTex = Material.GetTexture("_MainTex"); _MainTexScale = Material.GetTextureScale("_MainTex"); _MainTexOffset = Material.GetTextureOffset("_MainTex"); _NormalStrength = Material.GetFloat("_NormalStrength"); _NormalTex = Material.GetTexture("_NormalTex"); _NormalTexScale = Material.GetTextureScale("_NormalTex"); _NormalTexOffset = Material.GetTextureOffset("_NormalTex"); _EmColor = Material.GetColor("_EmColor"); _EmTex = Material.GetTexture("_EmTex"); _EmPower = Material.GetFloat("_EmPower"); _Smoothness = Material.GetFloat("_Smoothness"); _IgnoreDiffuseAlphaForSpeculars = Material.IsKeywordEnabled("FORCE_SPEC"); _RampChoice = (_RampInfoEnum)Material.GetFloat("_RampInfo"); _DecalLayer = (_DecalLayerEnum)Material.GetFloat("_DecalLayer"); _SpecularStrength = Material.GetFloat("_SpecularStrength"); _SpecularExponent = Material.GetFloat("_SpecularExponent"); _Cull_Mode = (_CullEnum)Material.GetFloat("_Cull"); _EnableDither = Material.IsKeywordEnabled("DITHER"); _FadeBias = Material.GetFloat("_FadeBias"); _EnableFresnelEmission = Material.IsKeywordEnabled("FRESNEL_EMISSION"); _FresnelRamp = Material.GetTexture("_FresnelRamp"); _FresnelPower = Material.GetFloat("_FresnelPower"); _FresnelMask = Material.GetTexture("_FresnelMask"); _FresnelBoost = Material.GetFloat("_FresnelBoost"); _EnablePrinting = Material.IsKeywordEnabled("PRINT_CUTOFF"); _SliceHeight = Material.GetFloat("_SliceHeight"); _PrintBandHeight = Material.GetFloat("_SliceBandHeight"); _PrintAlphaDepth = Material.GetFloat("_SliceAlphaDepth"); _PrintAlphaTexture = Material.GetTexture("_SliceAlphaTex"); _PrintAlphaTextureScale = Material.GetTextureScale("_SliceAlphaTex"); _PrintAlphaTextureOffset = Material.GetTextureOffset("_SliceAlphaTex"); _PrintColorBoost = Material.GetFloat("_PrintBoost"); _PrintAlphaBias = Material.GetFloat("_PrintBias"); _PrintEmissionToAlbedoLerp = Material.GetFloat("_PrintEmissionToAlbedoLerp"); _PrintDirection = (_PrintDirectionEnum)Material.GetFloat("_PrintDirection"); _PrintRamp = Material.GetTexture("_PrintRamp"); _EliteBrightnessMin = Material.GetFloat("_EliteBrightnessMin"); _EliteBrightnessMax = Material.GetFloat("_EliteBrightnessMax"); _EnableSplatmap = Material.IsKeywordEnabled("SPLATMAP"); _UseVertexColorsInstead = Material.IsKeywordEnabled("USE_VERTEX_COLORS"); _BlendDepth = Material.GetFloat("_Depth"); _SplatmapTex = Material.GetTexture("_SplatmapTex"); _SplatmapTexScale = Material.GetTextureScale("_SplatmapTex"); _SplatmapTexOffset = Material.GetTextureOffset("_SplatmapTex"); _SplatmapTileScale = Material.GetFloat("_SplatmapTileScale"); _GreenChannelTex = Material.GetTexture("_GreenChannelTex"); _GreenChannelNormalTex = Material.GetTexture("_GreenChannelNormalTex"); _GreenChannelSmoothness = Material.GetFloat("_GreenChannelSmoothness"); _GreenChannelBias = Material.GetFloat("_GreenChannelBias"); _BlueChannelTex = Material.GetTexture("_BlueChannelTex"); _BlueChannelNormalTex = Material.GetTexture("_BlueChannelNormalTex"); _BlueChannelSmoothness = Material.GetFloat("_BlueChannelSmoothness"); _BlueChannelBias = Material.GetFloat("_BlueChannelBias"); _EnableFlowmap = Material.IsKeywordEnabled("FLOWMAP"); _FlowTexture = Material.GetTexture("_FlowTex"); _FlowHeightmap = Material.GetTexture("_FlowHeightmap"); _FlowHeightmapScale = Material.GetTextureScale("_FlowHeightmap"); _FlowHeightmapOffset = Material.GetTextureOffset("_FlowHeightmap"); _FlowHeightRamp = Material.GetTexture("_FlowHeightRamp"); _FlowHeightRampScale = Material.GetTextureScale("_FlowHeightRamp"); _FlowHeightRampOffset = Material.GetTextureOffset("_FlowHeightRamp"); _FlowHeightBias = Material.GetFloat("_FlowHeightBias"); _FlowHeightPower = Material.GetFloat("_FlowHeightPower"); _FlowEmissionStrength = Material.GetFloat("_FlowEmissionStrength"); _FlowSpeed = Material.GetFloat("_FlowSpeed"); _MaskFlowStrength = Material.GetFloat("_FlowMaskStrength"); _NormalFlowStrength = Material.GetFloat("_FlowNormalStrength"); _FlowTextureScaleFactor = Material.GetFloat("_FlowTextureScaleFactor"); _EnableLimbRemoval = Material.IsKeywordEnabled("LIMBREMOVAL"); MaterialName = ((Object)Material).name; } } public void Update() { //IL_007c: Unknown result type (might be due to invalid IL or missing references) //IL_00ba: Unknown result type (might be due to invalid IL or missing references) //IL_00d1: Unknown result type (might be due to invalid IL or missing references) //IL_013d: Unknown result type (might be due to invalid IL or missing references) //IL_0154: Unknown result type (might be due to invalid IL or missing references) //IL_0182: Unknown result type (might be due to invalid IL or missing references) //IL_0427: Unknown result type (might be due to invalid IL or mi